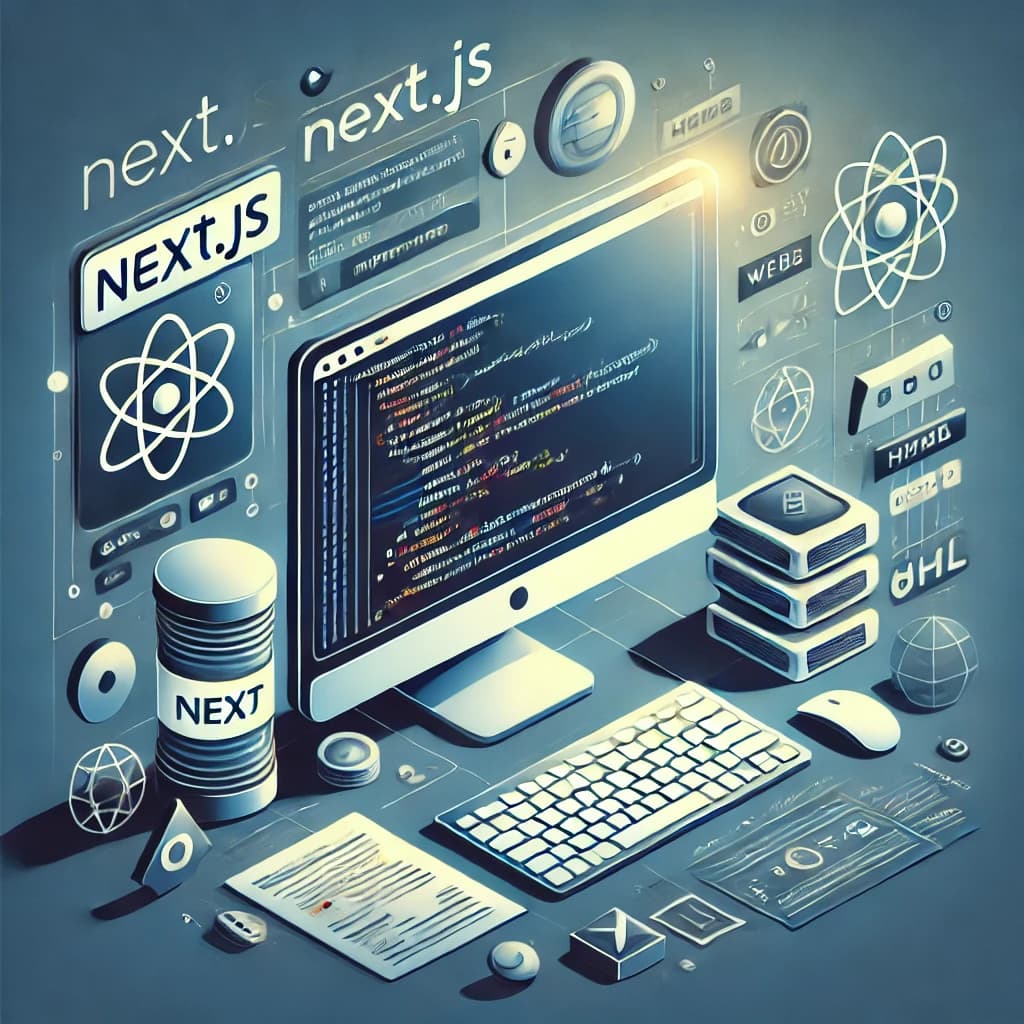
Introduction to Next.js
Brad Ivinson / October 9, 2024
Introduction to Next.js
Next.js is a powerful React framework that enables developers to build server-side rendered and statically generated web applications with ease. It provides a range of features that make it an excellent choice for modern web development. In this blog post, we'll explore the key features of Next.js and how you can get started with it.
What is Next.js?
Next.js is a React framework developed by Vercel that allows you to build fast and scalable web applications. It provides a hybrid static and server rendering, TypeScript support, smart bundling, route pre-fetching, and more. With Next.js, you can create both static websites and dynamic web applications.
Key Features of Next.js
Server-Side Rendering (SSR)
Next.js allows you to render your React components on the server side. This can improve the performance and SEO of your web application by delivering fully rendered HTML to the client.
Static Site Generation (SSG)
With Next.js, you can generate static HTML pages at build time. This is useful for creating fast, SEO-friendly websites that don't require server-side rendering for every request.
API Routes
Next.js provides a way to create API endpoints within your application. This means you can handle backend logic and database interactions directly within your Next.js project.
File-based Routing
Next.js uses a file-based routing system, which means the structure of your files and folders determines the routes of your application. This makes it easy to create and manage routes.
Automatic Code Splitting
Next.js automatically splits your code into smaller bundles, which can improve the performance of your application by loading only the necessary code for each page.
Built-in CSS and Sass Support
Next.js has built-in support for CSS and Sass, allowing you to style your components easily. You can also use CSS-in-JS libraries like styled-components or emotion.
Getting Started with Next.js
To get started with Next.js, you'll need to have Node.js and npm (or yarn) installed on your machine. Follow these steps to create a new Next.js project:
Create a New Next.js Project
Open your terminal and run the following command to create a new Next.js project:
npx create-next-app@latest my-next-app
Replace my-next-app
with the name of your project.
Navigate to Your Project Directory
cd my-next-app
Start the Development Server
npm run dev
This will start the development server, and you can view your application by navigating to http://localhost:3000
in your browser.
Creating Your First Page
Next.js uses a file-based routing system, so creating a new page is as simple as adding a new file to the pages
directory. Let's create a new page called about.js
.
Create the about.js
File
In the pages
directory, create a new file called about.js
and add the following code:
export default function About() {
return (
<div>
<h1>About Us</h1>
<p>Welcome to the about page!</p>
</div>
);
}
Navigate to the About Page
Open your browser and go to http://localhost:3000/about
to see your new page.
Conclusion
Next.js is a powerful framework that simplifies the process of building React applications with server-side rendering and static site generation. Its features, such as file-based routing, API routes, and automatic code splitting, make it an excellent choice for modern web development. By following the steps outlined in this blog post, you can get started with Next.js and begin building your own web applications.
Happy coding!