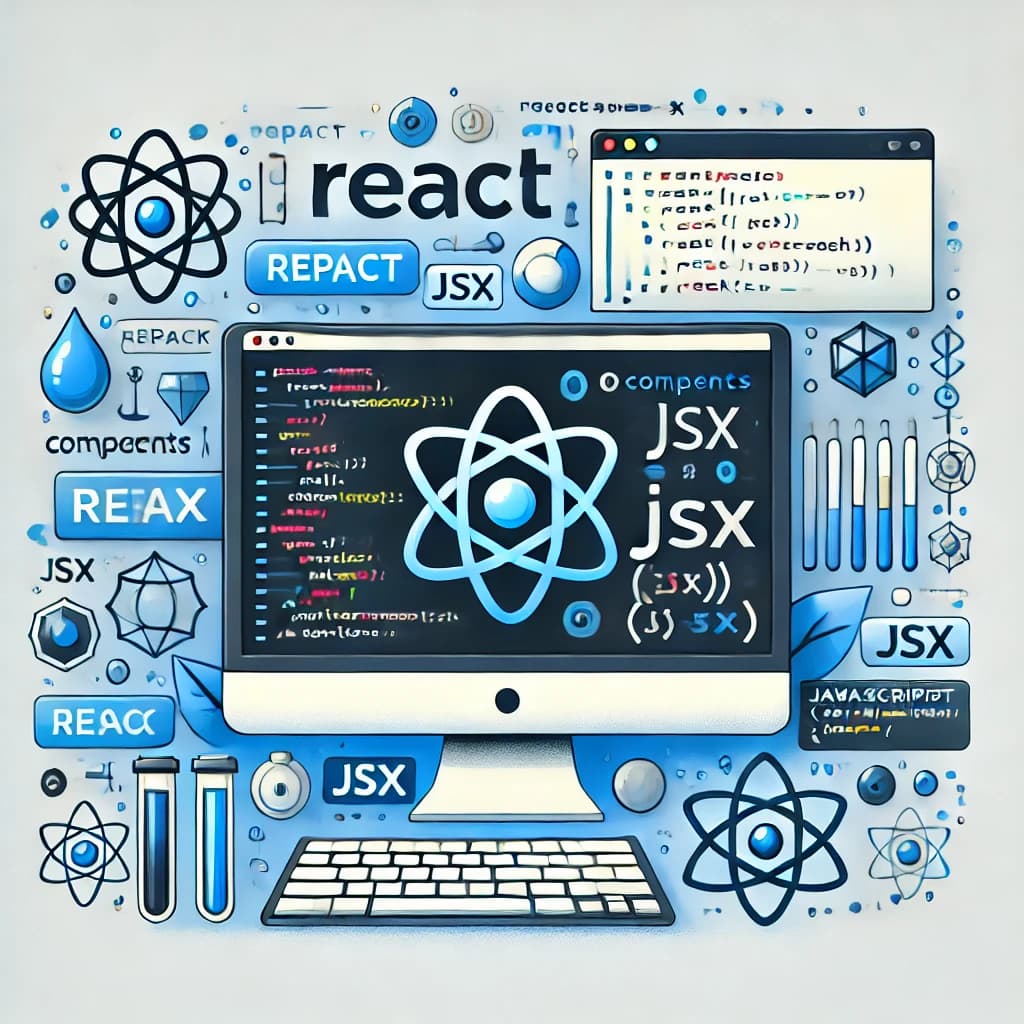
Getting Started with React
/ October 11, 2024
Getting Started with React
React is a popular JavaScript library for building user interfaces, particularly single-page applications where you need a fast, interactive user experience. Developed and maintained by Facebook, React has become one of the most widely used libraries in the web development community. In this blog post, we'll explore the basics of React and how you can get started with it.
What is React?
React is a JavaScript library for building user interfaces. It allows developers to create reusable UI components, manage the state of their applications, and efficiently update and render components when data changes. React follows a declarative programming paradigm, making it easier to reason about your application and predict its behavior.
Key Features of React
Component-Based Architecture
React encourages building applications using a component-based architecture. Components are the building blocks of a React application, and they can be reused and composed to create complex UIs. Each component manages its own state and can be nested within other components.
Virtual DOM
React uses a virtual DOM to optimize rendering performance. When the state of a component changes, React updates the virtual DOM first, then calculates the most efficient way to update the real DOM. This minimizes the number of DOM manipulations and improves the performance of your application.
JSX
JSX is a syntax extension for JavaScript that allows you to write HTML-like code within your JavaScript files. It makes it easier to create and visualize the structure of your components. JSX is compiled to JavaScript by tools like Babel.
Unidirectional Data Flow
React follows a unidirectional data flow, meaning data flows in one direction from parent components to child components. This makes it easier to understand how data changes affect your application and helps to maintain a predictable state.
Getting Started with React
To get started with React, you'll need to have Node.js and npm (or yarn) installed on your machine. Follow these steps to create a new React project:
Create a New React Project
Open your terminal and run the following command to create a new React project using Create React App:
npx create-react-app my-react-app
Replace my-react-app
with the name of your project.
Navigate to Your Project Directory
cd my-react-app
Start the Development Server
npm start
This will start the development server, and you can view your application by navigating to http://localhost:3000
in your browser.
Creating Your First Component
Let's create a simple React component called HelloWorld.js
.
Create the HelloWorld.js
File
In the src
directory, create a new file called HelloWorld.js
and add the following code:
import React from 'react';
function HelloWorld() {
return (
<div>
<h1>Hello, World!</h1>
<p>Welcome to your first React component.</p>
</div>
);
}
export default HelloWorld;
Use the HelloWorld
Component
Open src/App.js
and import the HelloWorld
component. Then, use it within the App
component:
import React from 'react';
import HelloWorld from './HelloWorld';
function App() {
return (
<div className="App">
<HelloWorld />
</div>
);
}
export default App;
View Your Component
Open your browser and go to http://localhost:3000
to see your new component in action.
Conclusion
React is a powerful library for building user interfaces, offering a component-based architecture, virtual DOM, JSX, and unidirectional data flow. By following the steps outlined in this blog post, you can get started with React and begin building your own web applications.
Happy coding!